Description
Castor3D is a 3D engine written in C++ 17.
It works on Windows and on GNU/Linux.
It uses Vulkan as rendering API.
The project itself is generable for supported platforms, using CMake.
It works on Windows and on GNU/Linux.
It uses Vulkan as rendering API.
Features
- Clustered lighting to compute all light sources.
- Using a visibility buffer for opaque objects.
- Weighted Blended rendering for transparent objects.
- Normal mapping (using Mikktspace or explicit bitangents specification).
- Shadow Mapping (allowing to choose between Raw, PCF or Variance Shadow Maps).
- Parallax Occlusion mapping.
- Screen Space Ambient Occlusion (using Scalable Ambiant Obscurance implementation).
- Reflection/Refraction Mapping.
- PBR rendering (hybrid Metallic/Specular/Roughness workflow), and Phong/Blinn-Phongrendering.
- HDR rendering with various tone mapping operators and various colour grading operators.
- Screen Space Subsurface Scattering (without backlit transmittance yet).
- Volumetric Light Scattering, for the directional light source.
- Cascaded Shadow Maps for the directional light source.
- Global Illumination, through Light Propagation Volules or Voxel Cone Tracing, your choice.
- Frustum culling.
- Scene graph.
- Render graph.
- Modular architecture through plug-ins.
- Shaders are generated automatically from material and pass configuration.
- Shaders are writable directly from C++ code.
- Scenes are described using a text format easily comprehensible and extensible.
- Asynchronous or synchronous rendering.
- Using Mesh and Task shaders, if available.
- GUI primitives.
Implemented plugins
Importers
Importer plugins can add support for new mesh file formats.- ASSIMP: Multiple format mesh importer, using assimp library.
- glTF: glTF 2.0 importer, more precise than assimp's provided one, using fastgltf library.
PostEffects
- Bloom: HDR Bloom implementation.
- PbrBloom: PBR Bloom implementation.
- DrawEdges: Detects and renders edges, based on normal, depth, and or object ID.
- FilmGrain: To display some grain on the render.
- GrayScale: Converts render in gray scale.
- LightStreaks (using Kawase Light Streaks).
- FXAA Antialiasing.
- SMAA Antialiasing (1X and T2X so far).
- Linear Motion Blur.
- DepthOfField: Depth of Field implementation.
Generators
They allow procedural generation of meshes or textures.- DiamondSquareTerrain: to generate terrains inside Castor3D scenes, using diamond-quare algorithm.
Generics
They're here to allow general extensions of the engine features.- ToonMaterial: A toon material (to be combined with DrawEdges plugin).
- WaterMaterial: Water material, using normal maps.
- FFTOceanRendering: Ocean rendering using FFT generated surfaces.
- WavesRendering: Basic ocean rendering, specifying waves attributes.
- AtmosphereScattering : Sky and atmosphere rendering.
ToneMappings
The definitions of tone mapping operators.- None: A passthrough, used when rendering to HDR screen.
- Linear: Default tone mapping.
- Haarm Pieter Duiker.
- Hejl Burgess Dawson (Filmicx).
- Reinhard.
- Uncharted 2.
- ACES.
Other applications
Along with the engine and its plugins, the projects also comes with other applications:- CastorViewer, a scene visualizer, using Castor3D.
- ImgConverter, image converter, from all images formats to XPM or ICO.
- CastorMeshConverter: A converter from various mesh files to Castor3D mesh format.
- CastorMeshUpgrader: Upgrades from earlier versions of Castor3D mesh format to the latest one.
- HeightMapToNormalMap: Converts a height map to a normal map.
Documentation
The project's documentation is contained in the headers, and can be generated with Doxygen.The project itself is generable for supported platforms, using CMake.
Examples
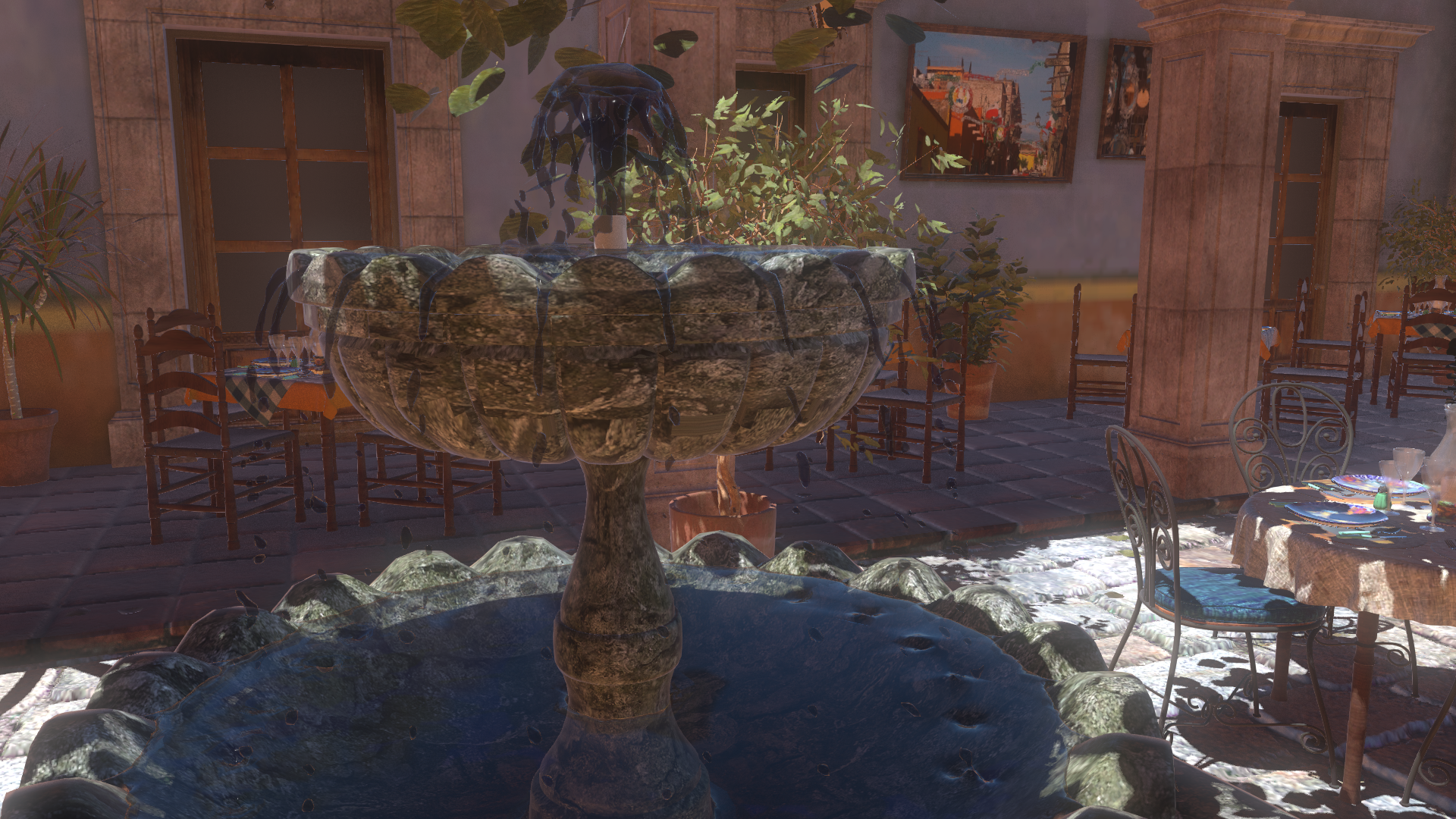
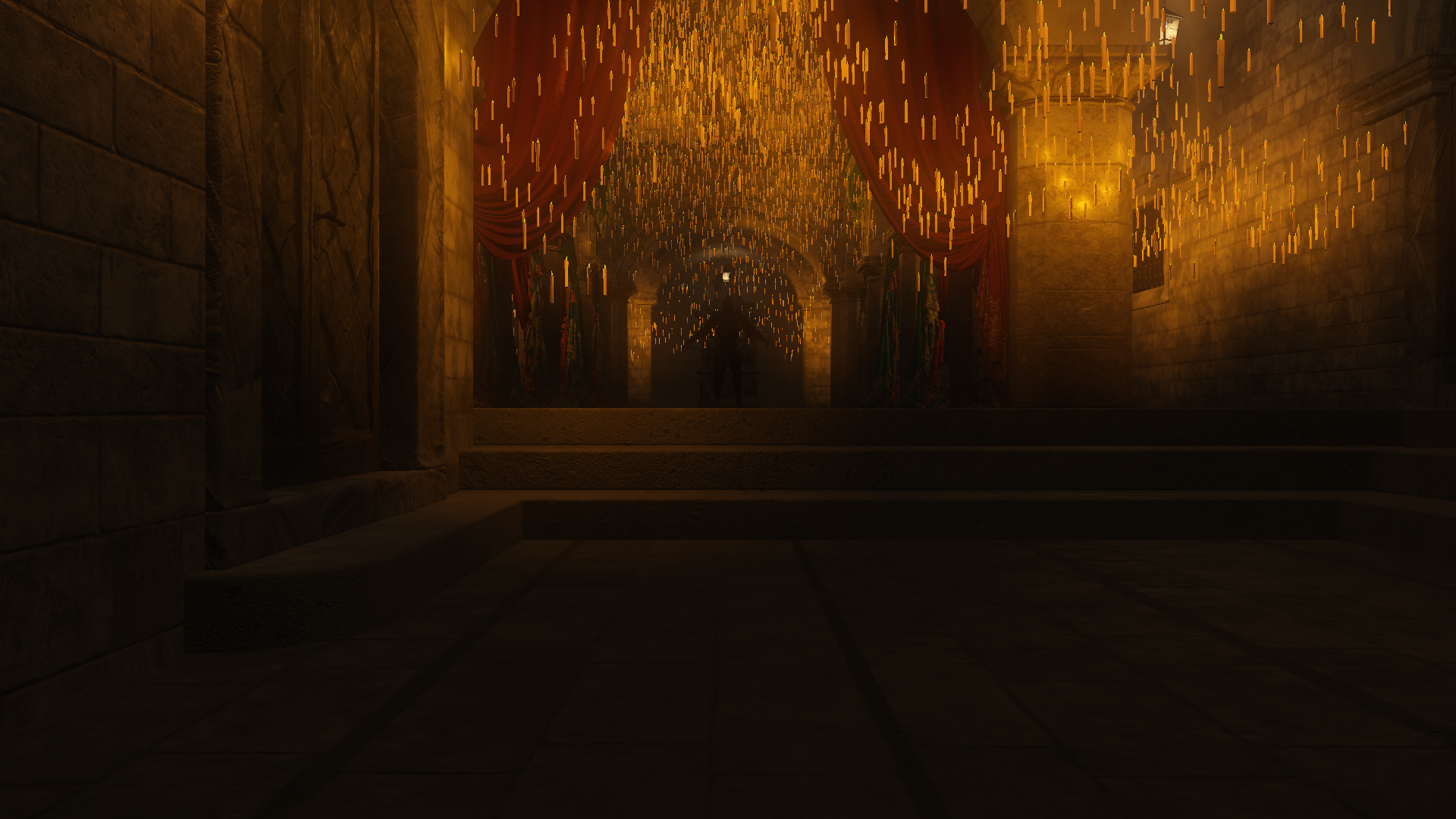
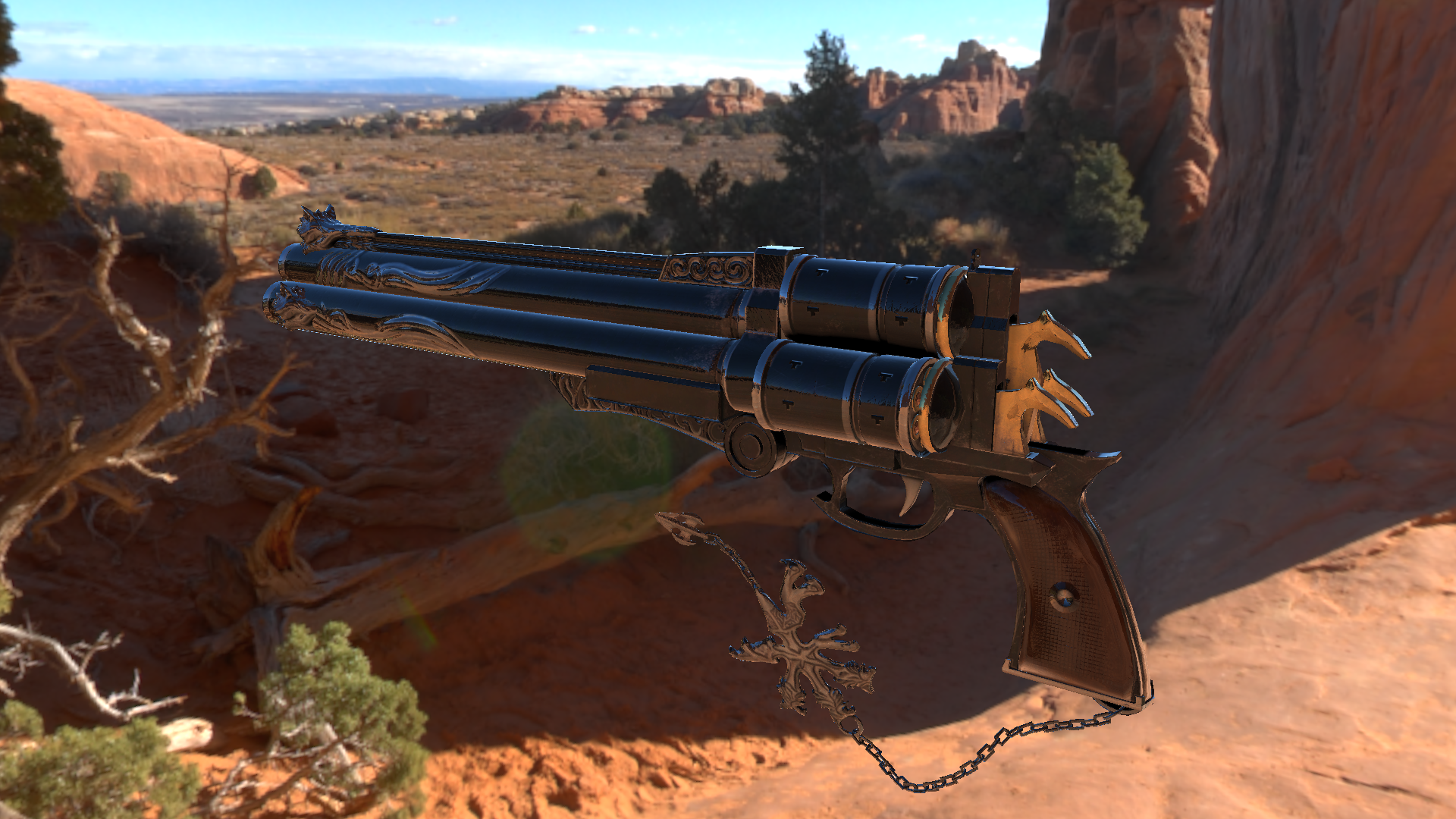
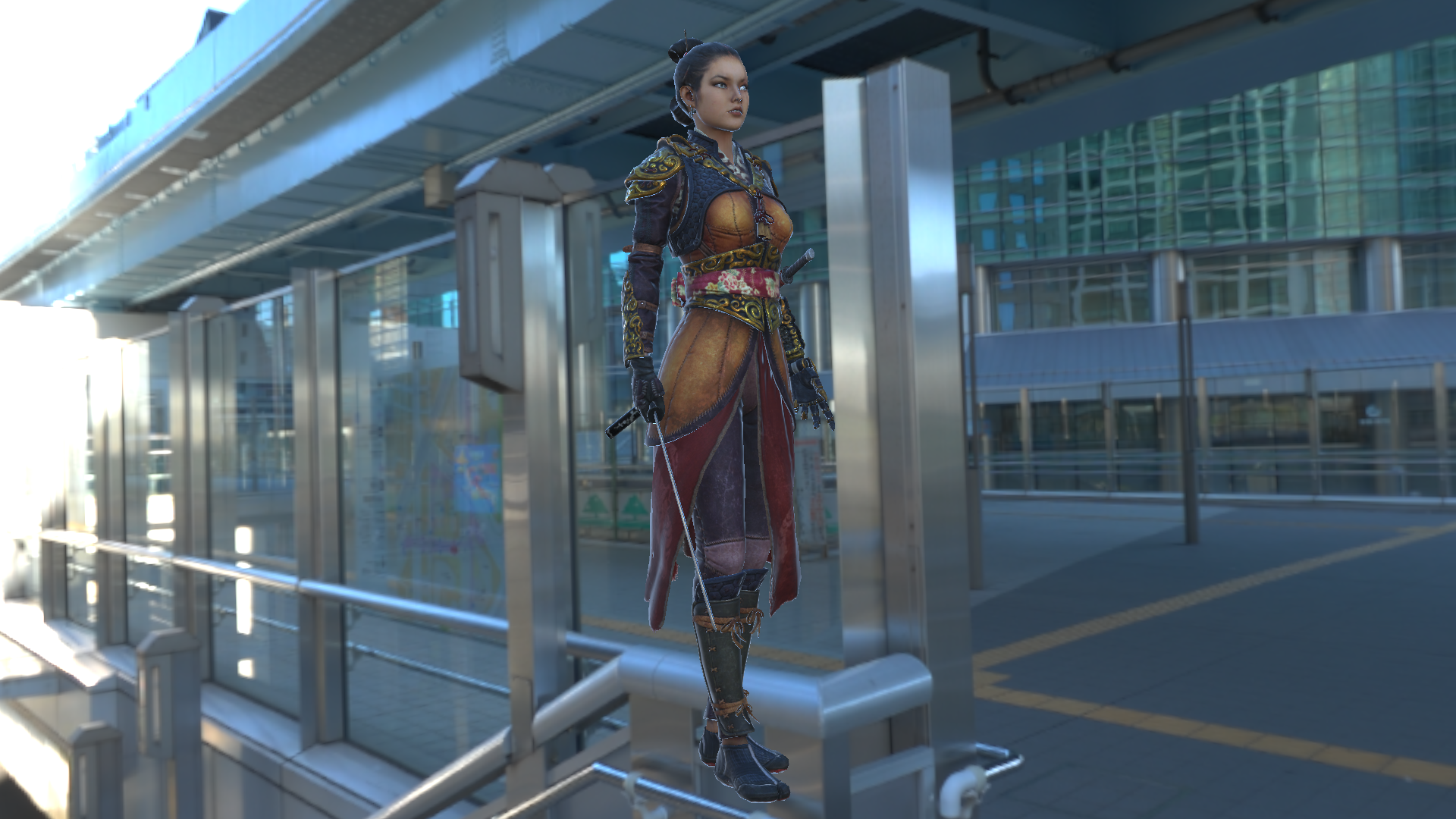
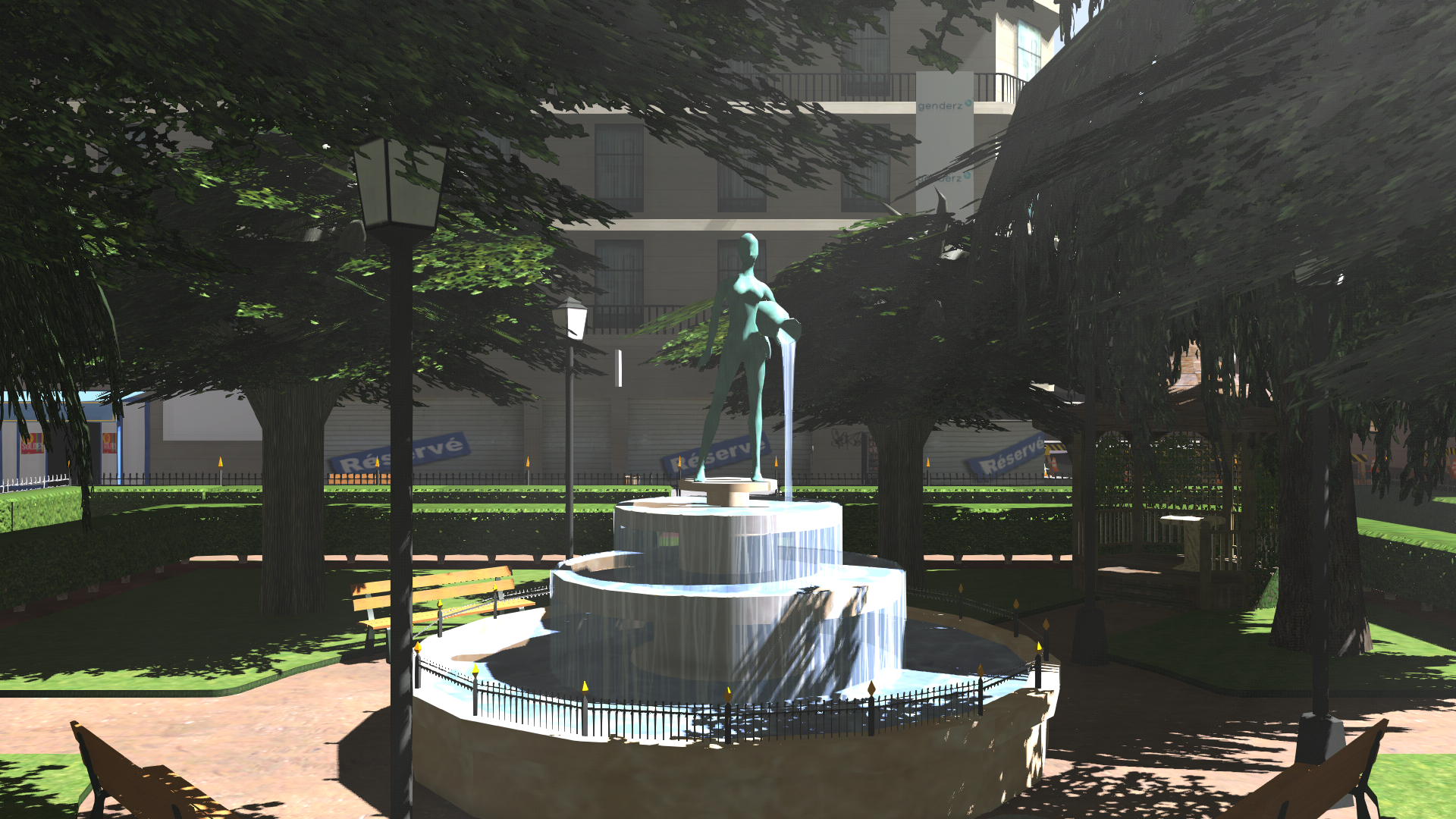

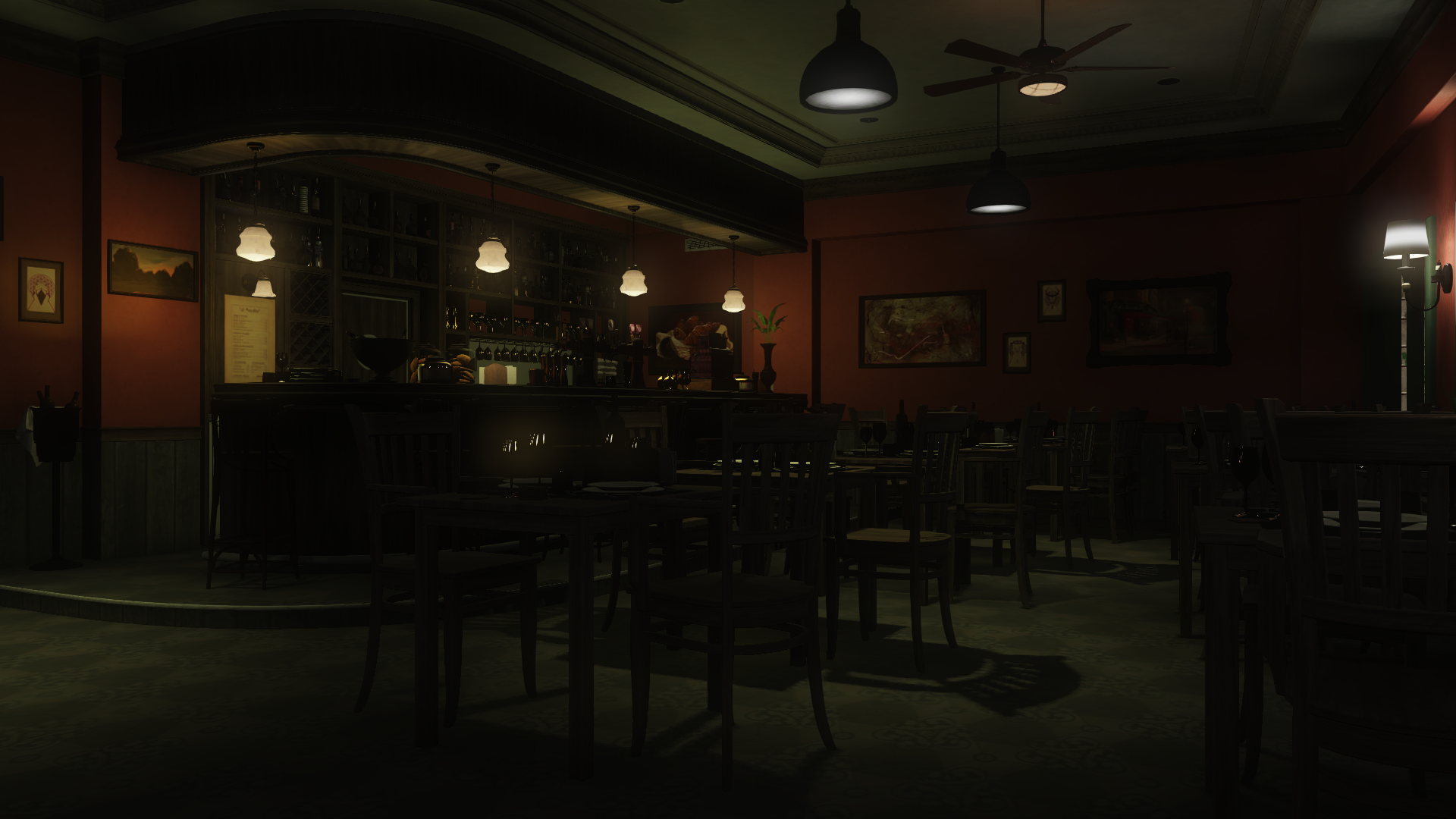
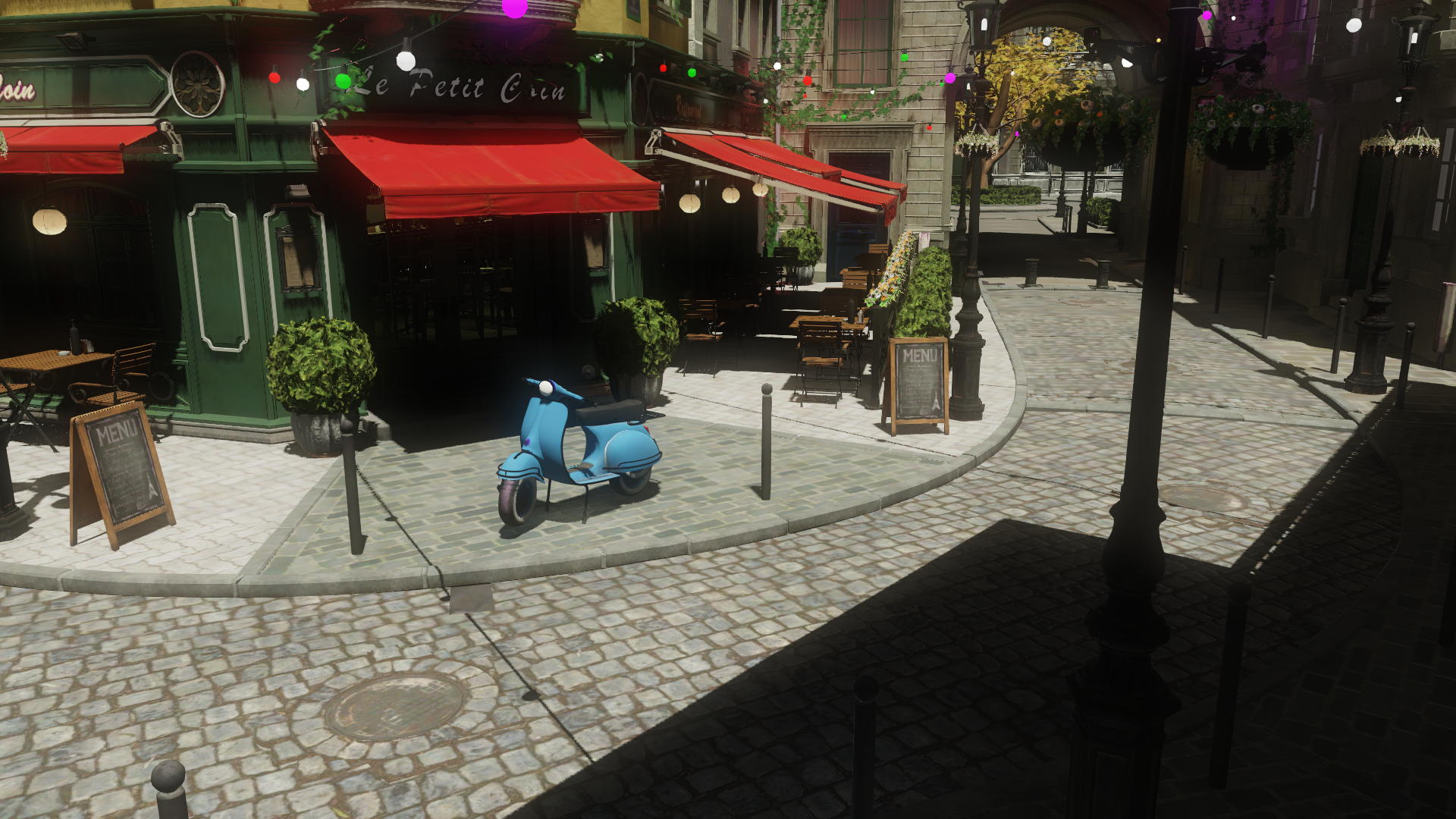
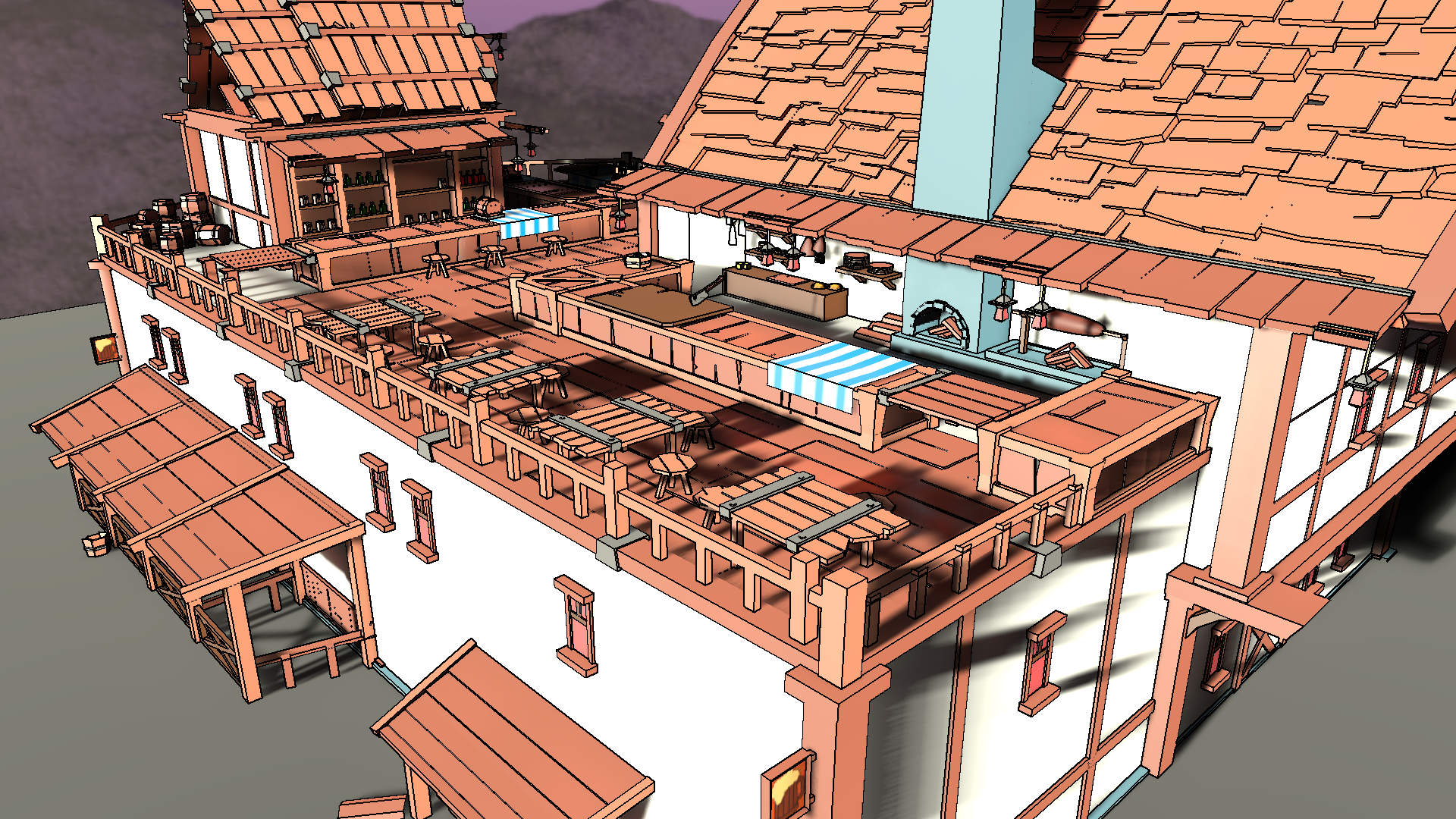

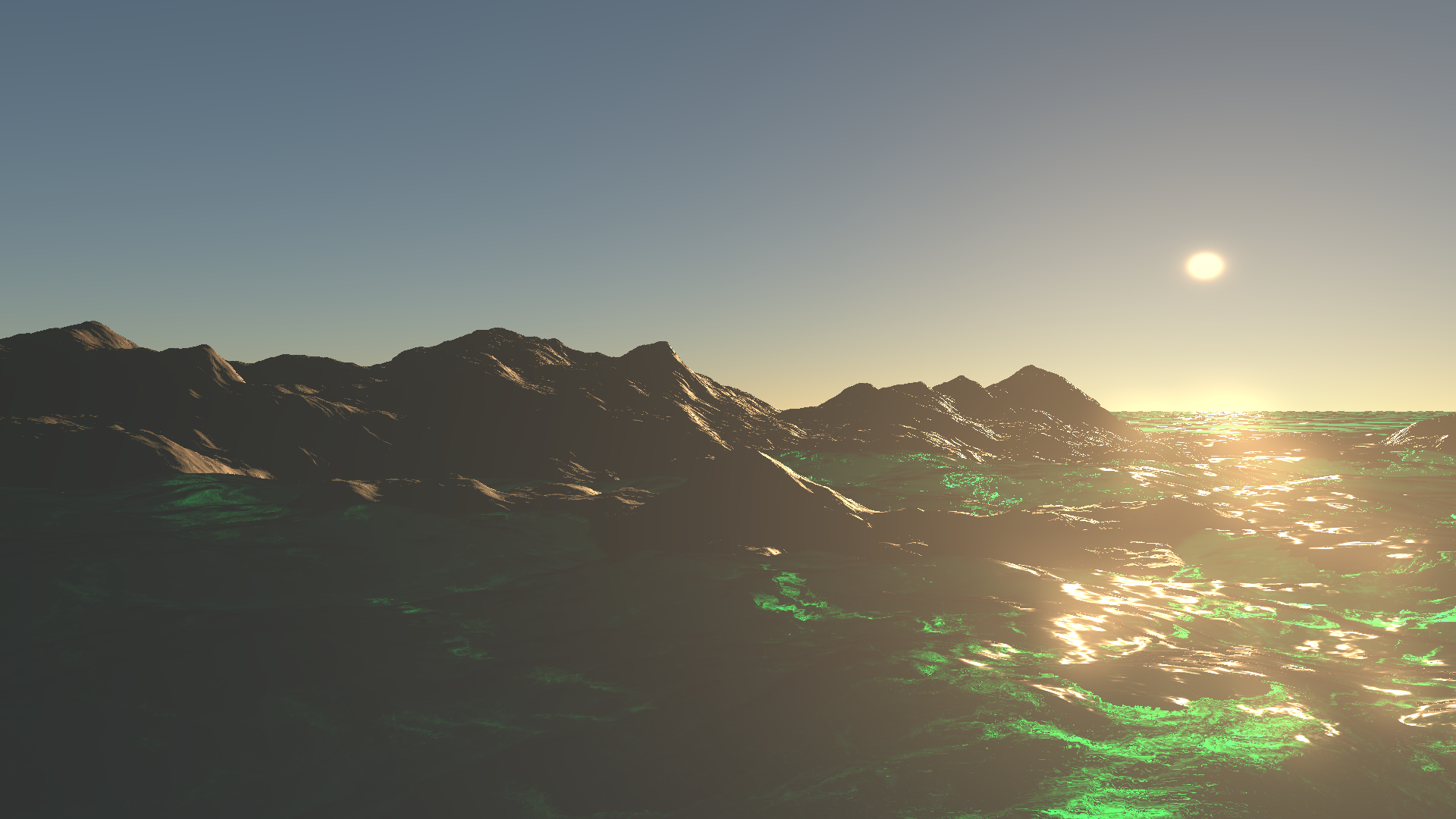